test-37 hackerrank solution 1) #include <stdio.h> #include <string.h> #include <math.h> #include <stdlib.h> int main() { /* Enter your code here. Read input from STDIN. Print output to STDOUT */ int n, i; scanf("%d", &n); int s[n+30]; for (i = 0; i < n; i++) scanf("%d", &s[i]); double change, weekdaychange[7] = { 0 }; int weekdaycount[7] = { 0 }; for (i = 1; i < n; i++) { change = (s[i] - s[i-1]) * 100 / (float)s[i-1]; if (abs(change) < 40) { weekdaychange[i%7] += change; weekdaycount[i%7]++; } } for (i = 0; i < 7; i++) weekdaychange[i] /= weekdaycount[i]; weekdaychange[0] *= 0.6; //weekday percentage sign uncertainty coef depending also on the magnitude of the percentage value weekdaychange[1] *= 0.6; weekdaychange[2] *= 0.6; weekdaychange[3] *= 0.3; weekdaychange[4] *= 0....
Posts
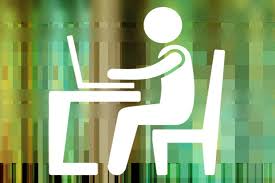
test-38 solution hackerrank 1) #include <stdio.h> #define P 1000000007LL long long I,g,b[1000], r,h,a[2000000],i,j,k,l,m,n, s[2000000],t,min, v[200000]; long long obsah(long long nn) { long long vv=1, ii; for(ii=0;ii<k;ii++) vv = (vv*(b[ii]/nn))%P; return vv; } long long next(long long jj) { long long kk,ii, ne; kk = b[0]/jj; ne = b[0]/kk + 1; for(ii=1;ii<k;ii++) { kk = b[ii]/jj; if(ne > b[ii]/kk +1) ne = b[ii]/kk + 1; } return ne; } int main() { scanf("%lld",&t); while(t) { t--; scanf("%lld",&k); for(i=0;i<k;i++) scanf("%lld",b+i); min = b[0]; for(i=0;i<k;i++) if(min>b[i]) min = b[i]; //printf("zac\n"); //a[0] = 1; //s[0] = obsah[1]; j = 1; l = 0; while(1) { //printf("%lld =j\n",j); a[l] = j; s[l] = obsah(j); l++; if(j>min) break; j = next(j); } for(m=l-1;m>=0;m--) { v[m] = s[m]; i = 2; h = 1; ...
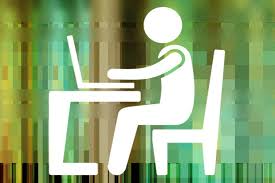
test-36 solutions hacker rank LINK: https://www.hackerrank.com/contests/ececodetest36 1) #include <stdio.h> #include <math.h> int n; double ans = -1e100; double mx[3000010],mn[3000010]; int main() { int i; double v; mx[0] = mn[0] = cos(0); mx[1] = mn[1] = cos(0.5); for(i=2;i<=3000000;i++) { v = cos(i/2.0); mx[i] = v > mx[i-2] ? v : mx[i-2]; mn[i] = v < mn[i-2] ? v : mn[i-2]; } scanf("%d",&n); for(i=1;i<=n-2;i++) { v = 2*sin((n-i)/2.0); v *= v > 0 ? mx[n-i-2] : mn[n-i-2]; v += sin(i); ans = ans > v ? ans : v; } printf("%.9f",ans); return 0; } 2) #include <stdio.h> #include <stdlib.h> #include <math...
1) #include <stdio.h> #include <string.h> #include <math.h> #include <stdlib.h> unsigned long max_sum( unsigned long *partial_sums, unsigned long *dest, unsigned length, unsigned long target ) { if (length < 2) return (*dest = *partial_sums); unsigned lower_len = length >> 1, upper_len = length - lower_len; unsigned long lower[lower_len], upper[upper_len], max_left = max_sum(partial_sums, lower, lower_len, target), max_right = max_sum(&partial_sums[lower_len], upper, upper_len, target), max = max_left > max_right ? max_left : max_right; unsigned lesser = 0, greater = 0; while (lesser < lower_len && greater < upper_len) if (upper[greater] < lower[lesser]) { *dest = upper[greater++]; unsigned long other_max = (*dest++ - lower[lesser]) + target; if (other_max > max) ...
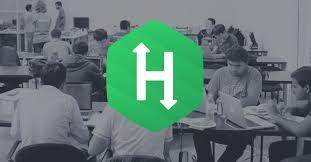
Hackerrank test-28 solutions programs Link: https://www.hackerrank.com/contests/skd-ece-28 2) #include <stdio.h> #include <stdlib.h> int mod=1000000007; int fact(int n) { return (n <= 1)? 1 :(n * fact(n-1))%mod; } int findSmallerInRight(int* str, int low, int high) { int countRight = 0, i; for (i = low+1; i <= high; ++i) if (str[i] < str[low]) ++countRight; return countRight; } int findRank (int* str,int n) { int len = n; int mul ; int rank = 1; int countRight; int i; for (i = 0; i < len; ++i) { mul = fact(len - i - 1); countRight = findSmallerInRight(str, i, len-1); rank = (rank + countRight * mul)%mod ; } return rank; } void swap(int *a,int *b) { int temp; temp=*a; *a=*b; *b=temp; } void heapPermutation(int a[], int size, int n,int c[],int m,int d[],int *S) ...
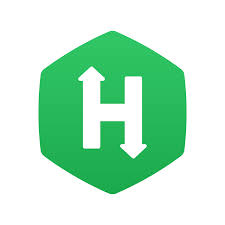
hacker rank Test Solutions-26 LINK: https://www.hackerrank.com/contests/ece-coding-test-26 1) #include <stdio.h> #include <string.h> #include <stdlib.h> #include <malloc.h> typedef long long LLG; #ifndef DEBUG static char* debug; #endif int LLGqsort( const void* lArg, const void* rArg ) { LLG* lhs = (LLG*) lArg; LLG* rhs = (LLG*) rArg; # ifndef DEBUG if (debug) fprintf(stderr,"."); # endif return *lhs<*rhs ? -1 : (*lhs>*rhs ? 1 : 0); } LLG LLGGCD( LLG a, LLG b) { if (!b) return a; return LLGGCD( b, a%b ); } int main() { LLG N, K, Ksave, Fs[100][2], f, df; LLG U; int nFs = 0; int iFs; int iUs; int dumy; LLG n; LLG nDivs; LLG *pDiv0, *pDivEnd, *pDiv; LLG step; LLG gcd; # ifndef DEBUG debug = getenv( "DEBUG" ); # endif dumy = fscanf( stdin, "%Ld", &N); dumy = fscanf( stdin, "%Ld", &K); Ksave = K; memset( Fs, 0, sizeof Fs ); if ( K==1LL ) { ...
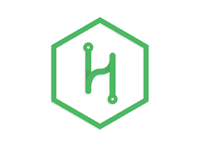
Hacker rank test-25 solutions Test-25 solutions LINK: https://www.hackerrank.com/contests/ece-coding-test-25 1) #include <math.h> #include <stdio.h> #define READNUM 9 double one_twochar(char input2[READNUM]){ return(0.0); } double threechar(char input2[READNUM]){ if(input2[0]==input2[2]){ return(0.0); } else{ return(3.0); } } double fourchar(char input2[READNUM]){ if(input2[0]==input2[3]){ return(0.0); } else{ return(3.0); } } double five_aabbc(char input2[READNUM],char center){ char chartemp; int i; if(input2[2]==center){ return (15.6250); } else{ for(i=0;i<5;i+=1){ if(input2[i]==center){ input2[i]=input2[2]; input2[2]=center; } } if((input2[0]==input2[4])&&(input2[1]==input2[3])){ return(19.375); } else{ return(20.9375); } } } double five_aaabb(char input2[READNUM],char ...
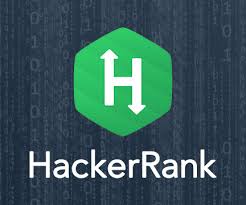
hackerrank test-20 solutions Test-20 SOLUTIONS 1) #include <stdio.h> #include <string.h> int is_a(const char* a, const char* b) { while (*a && *b) { if (*a == *b) { a++; b++; } else { return *a < *b; } } return *a != 0; } void merge(const char* a, const char* b, char* c) { int is_a_preferred = is_a(a, b); while (*a && *b) { if (*a == *b) { *c = (is_a_preferred)? *(a++) : *(b++); } else { *c = (*a < *b)? *(a++) : *(b++); is_a_preferred = is_a(a, b); } ++c; } while (*a) { *c++ = *a++; } while (*b) { *c++ = *b++; } *c = '\0'; } int main() { int t; char a[100001]; char b[100001]; char c[200001]; scanf("%d", &t); while (t--) { scanf("%s\n%s", a, b); merge(a, b, c); printf(...